Reading Time: 3 minutes
Aim
Last time I showed how I had written a control for inputting an angle. This time I am improving the look of the control as well as adding touch input.
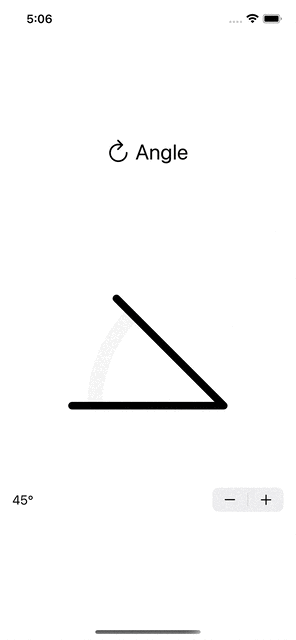
From CGFloat to Angle
But first I want to make an improvement to the code. Now the angle is stored as a CGFloat value with convenience functions to convert between radians and degrees. However, this means that it is up to me to remember in which unit the value is at any given moment. Turns out SwiftUI has a struct that solves this: Angle
. You instantiate it with Angle.degrees(90)
or Angle.radians(.pi)
and you can ask for the angle in either unit with .degrees and .radians.
Making Angle animatable
Now however, things get a bit weird. Sadly the animation has stopped working. Tapping on the stepper makes the line jump instead of animating. Turns out that while Angle conforms to the Animatable protocol itself, it doesn’t conform to the VectorArithmetic protocol. This is needed for the Shape to be animatable. Conforming to VectorArithmetic also means conforming to AdditiveArithmetic.
Luckily it is simple enough to implement.
And just by adding those two conformances the input animates again!
Drawing wedge shape
Next I want draw a wedge shape to fill the space between the two lines. To be able to draw this wedge in a different color I need to create a separate shape. Here having the angle in the Angle struct is convenient as that can be used in the call to draw a part of an arc.
Now I have two shapes: WedgeShape and AngleShape which can each have the desired fill or stroke colors and be stacked on top of each other using a ZStack.
Adding Touch Input
Having an input control that doesn’t take touch isn’t much of a control. Next up is adding the ability to use your finger to drag the angle.
A DragGesture can facilitate this. When the drag gesture recognizes a change I set the variable isEditing to true so that the view can show the user it is being manipulated. And more importantly, the new angle is calculated from the point of rotation to where the user touches the control. Here I am using atan2 which takes care of the division so you don’t have to worry about dividing by zero.
When the drag ends, the variable isEditing should be false again.
Now I am ready to add the gesture to the AngleView using the view modifier .gesture(dragGesture).
Now, while this works, it doesn’t feel very responsive. The UI feels laggy. This is because the change in angle is animated, so when changing the angle from the drag gesture animations should be disabled. To achieve this I wrapped the line where the angle is set in a Transaction which has animations disabled.
Editing mode
To let the user know the control is being edited I pass the value of the isEditing var to the AngleShape and draw a circle at the end of the path.
Code
You can find all the code created in this post on GitHub.

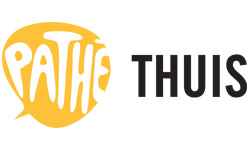
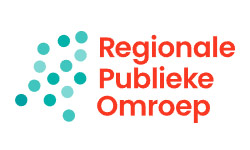
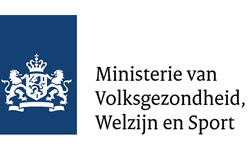
